Bot solutions on Microsoft Azure are supported by the following technologies:
Azure Bot Service. A cloud service that enables bot delivery through one or more channels, and integration with other services.
Bot Framework Service. A component of Azure Bot Service that provides a REST API for handling bot activities.
Bot Framework SDK. A set of tools and libraries for end-to-end bot development that abstracts the REST interface, enabling bot development in a range of programming languages.

Bot templates
The easiest way to get started with the Bot Framework SDK is to base your new bot on one the templates it provides:
Empty Bot - a basic bot skeleton.
Echo Bot - a simple "hello world" sample in which the bot responds to messages by echoing the message text back to the user.
Core Bot - a more comprehensive bot that includes common bot functionality, such as integration with the Language Understanding service.
Bot application classes and logic
The template bots are based on the Bot class defined in the Bot Framework SDK, which is used to implement the logic in your bot that receives and interprets user input, and responds appropriately. Additionally, bots make use of an Adapter class that handles communication with the user's channel.
Conversations in a bot are composed of activities, which represent events such as a user joining a conversation or a message being received. These activities occur within the context of a turn, a two-way exchange between the user and bot. The Bot Framework Service notifies your bot's adapter when an activity occurs in a channel by calling its Process Activity method, and the adapter creates a context for the turn and calls the bot's Turn Handler method to invoke the appropriate logic for the activity.
Testing with the Bot Framework Emulator
Bots developed with the Bot Framework SDK are designed to run as cloud services in Azure, but while developing your bot, you'll need a way to test it before you deploy it into production.
The Bot Framework Emulator is an application that enables you to run your bot a local or remote web application and connect to it from an interactive web chat interface that you can use to test your bot. Details of activity events are captured and shown in the testing interface, so you can monitor your bots behavior as you submit messages and review the responses.
Implement activity handlers and dialogs
The logic for processing the activity can be implemented in multiple ways. The Bot Framework SDK provides classes that can help you build bots that manage conversations using:
Activity handlers: Event methods that you can override to handle different kinds of activities.
Dialogs: More complex patterns for handling stateful, multi-turn conversations.
Activity handlers
For simple bots with short, stateless interactions, you can use Activity Handlers to implement an event-driven conversation model in which the events are triggered by activities such as users joining the conversation or a message being received. When an activity occurs in a channel, the Bot Framework Service calls the bot adapter's Process Activity function, passing the activity details. The adapter creates a turn context for the activity and passes it to the bot's turn handler, which calls the individual, event-specific activity handler.
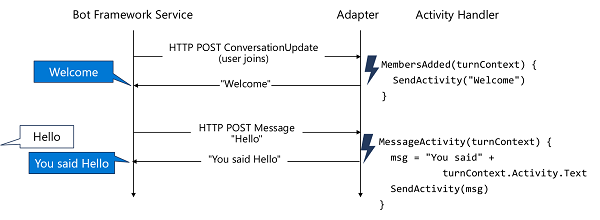
The ActivityHandler base class includes event methods for the many kinds of common activity, including:
Message received
Members joined the conversation
Members left the conversation
Message reaction received
Bot installed
Others...
You can override any activity handlers for which you want to implement custom logic.
Turn context
An activity occurs within the context of a turn, which represents a single two-way exchange between the user and the bot. Activity handler methods include a parameter for the turn context, which you can use to access relevant information. For example, the activity handler for a message received activity includes the text of the message.
More Information
For more information about activity handlers, see the Bot Framework SDK documentation.
Dialogs
For more complex conversational flows where you need to store state between turns to enable a multi-turn conversation, you can implement dialogs. The Bot Framework SDK dialogs library provides multiple dialog classes that you can combine to implement the required conversational flow for your bot.
There are two common patterns for using dialogs to compose a bot conversation:
1. Component dialogs
A component dialog is a dialog that can contain other dialogs, defined in its dialog set. Often, the initial dialog in the component dialog is a waterfall dialog, which defines a sequential series of steps to guide the conversation. It's common for each step to be a prompt dialog so that conversational flow consists of gathering input data from the user sequentially. Each step must be completed before passing the output onto the next step
For example, a pizza ordering bot might be defined as a waterfall dialog in which the user is prompted to select a pizza size, then toppings, and finally prompted for payment.
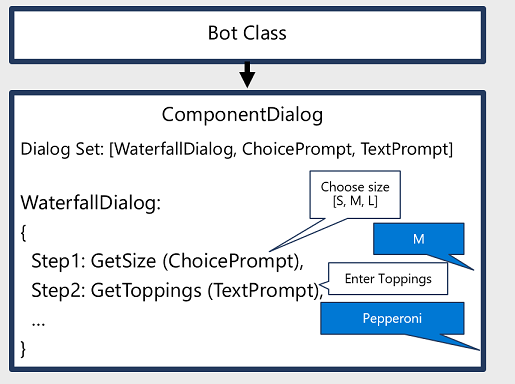
2. Adaptive dialogs
An adaptive dialog is another kind of container dialog in which the flow is more flexible, allowing for interruptions, cancellations, and context switches at any point in the conversation. In this style of conversation, the bot initiates a root dialog, which contains a flow of actions (which can include branches and loops), and triggers that can be initiated by actions or by a recognizer. The recognizer analyzes natural language input (usually using the Language Understanding service) and detects intents, which can be mapped to triggers that change the flow of the conversation - often by starting new child dialogs, which contain their own actions, triggers, and recognizers.
For example, the pizza ordering bot might start with a root dialog that simply welcomes the user. When the user enters a message indicating that they want to order a pizza, the recognizer detects this intent and uses a trigger to start another dialog containing the flow of actions required to gather information about the pizza order. At any point during the pizza order dialog, the user might enter a message indicating that they want to do something else (for example, cancel the order), and the recognizer for the pizza ordering dialog (or its parent dialog) can be used to trigger an appropriate change in the conversational flow.
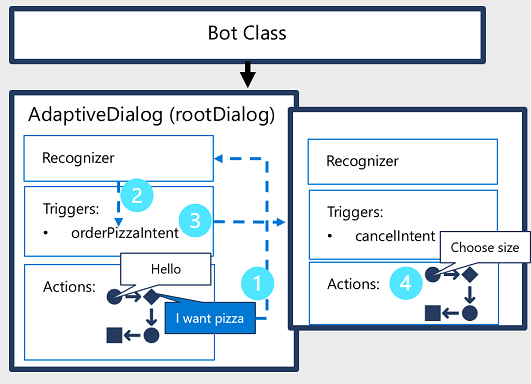
Note Using dialogs offers greater flexibility than is possible with a bot based purely on activity handlers, but can be more complex to program. While you can use the Bot Framework SDK to implement dialogs in code, you may want to consider using the Bot Framework Composer to build bots with complex dialogs, taking advantage of its visual design interface.
Deploy a bot
After you've completed development of your bot, you can deploy it to Azure. The specific details of how the bot is hosted varies, depending on the programming language and underlying runtime you have used; but the basic steps for deployment are the same.
Create the Azure resources required to support your bot
Your will need to create an Azure application registration to give your bot an identity it can use to access resources, and a bot application service to host the bot.
Register an Azure app
You can create the application registration by using the az ad app create Azure command-line interface (CLI) command, specifying a display name and password for your app identity. This command registers the app and returns its registration information, including a unique application ID that you will need in the following step.
Create a bot application service
Your bot requires a Bot Channels Registration resource, along with associated application service and application service plan. To create these resources, you can use the Azure resource deployment templates provided with the Bot Framework SDK template you used to create your bot. Just run the az deployment group create command, referencing the deployment template and specifying your bot application registration's ID (from the az ad app create command output) and the password you specified.
Prepare your bot for deployment
The specific steps you need to perform to prepare your bot depend on the programming language used to create it. For C# and JavaScript bots, you can use the az bot prepare-deploy command to ensure your bot is properly configured with the appropriate package dependencies and build files. For Python bots, you must include a requirements.txt file listing any package dependencies that must be installed in the deployment environment.
Deploy your bot as a web app
The final step is to package your bot application files in a zip archive, and use the az webapp deployment source config-zip command to deploy the bot code to the Azure resources you created previously.
After deployment has completed, you can test and configure your bot in the Azure portal.
More Information
For more information about deploying a bot, see the Bot Framework SDK documentation.
Tips for create chatbot by using Node JS
Call all the JavaScript classes in Index.js file
Create separate file for welcome message
Extend the welcome file to intend handle for handle the user intents
Create LUIS and dialogs for response for user queries
Comments